Inputs: ScriptSig Size
Size
variable
Format
Compact Size
Description
The size in bytes of the upcoming ScriptSig.
Example
6b
Byte Visualization
The ScriptSig size is stored as a variable-length integer (varint) that indicates the length of the following ScriptSig field in bytes.
Let's examine our transaction to understand this better:
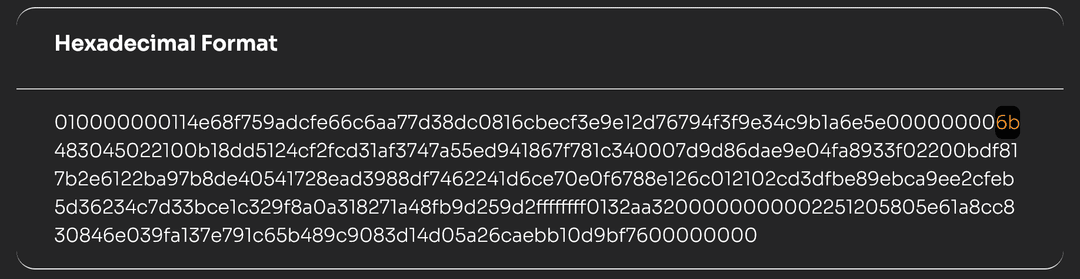
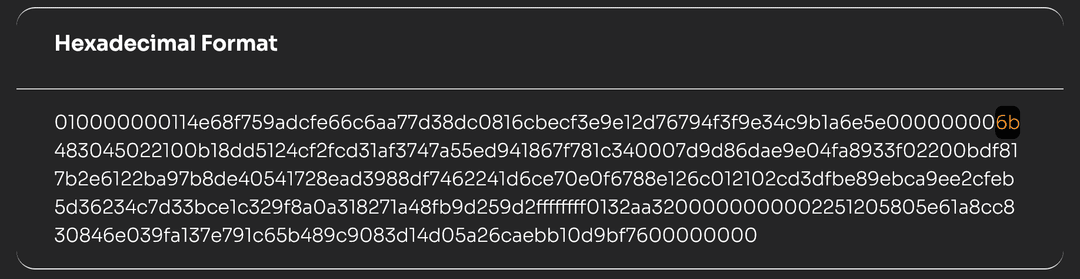
In this transaction, we can see the ScriptSig size value: 6b
. When decoded, this hexadecimal value represents 107 bytes, telling us that the following ScriptSig data will be exactly 107 bytes long.
Variable Integer Encoding
Like the input count, the ScriptSig size uses varint encoding:
Decimal Range | Hex Range | Bytes used | Format |
---|---|---|---|
0 to 252 | 0x00 to 0xfc | 1 | uint8_t |
253 to 2¹⁶-1 | 0xfd to 0xffff | 3 | 0xfd followed by uint16_t |
2¹⁶ to 2³²-1 | 0x10000 to 0xffffffff | 5 | 0xfe followed by uint32_t |
2³² to 2⁶⁴-1 | 0x100000000 to 0xffffffffffffffff | 9 | 0xff followed by uint64_t |
Note
Most ScriptSig sizes are small enough to fit in a single byte, as the ScriptSig typically contains just a signature and public key that combined are less than 253 bytes in size.
Implementation Example
Here's how you might parse a ScriptSig size:
def parse_scriptsig_size(raw_tx: bytes, offset: int = 0) -> tuple[int, int]:
"""
Parse ScriptSig size from raw transaction bytes
Args:
raw_tx: Raw transaction bytes
offset: Starting position in bytes
Returns:
(size, new_offset)
"""
# Read the varint
size = 0
first_byte = raw_tx[offset]
if first_byte < 0xfd:
size = first_byte
offset += 1
elif first_byte == 0xfd:
size = int.from_bytes(raw_tx[offset+1:offset+3], 'little')
offset += 3
elif first_byte == 0xfe:
size = int.from_bytes(raw_tx[offset+1:offset+5], 'little')
offset += 5
else: # 0xff
size = int.from_bytes(raw_tx[offset+1:offset+9], 'little')
offset += 9
return size, offset