Inputs: ScriptSig
Size
variable
Format
Script
Description
The unlocking script that fulfills the conditions of the output.
Example
483045022100...
Byte Visualization
The ScriptSig is a variable-length field in transaction inputs that provides the "unlocking" data needed to spend a previous output. Let's examine our transaction to understand this better:
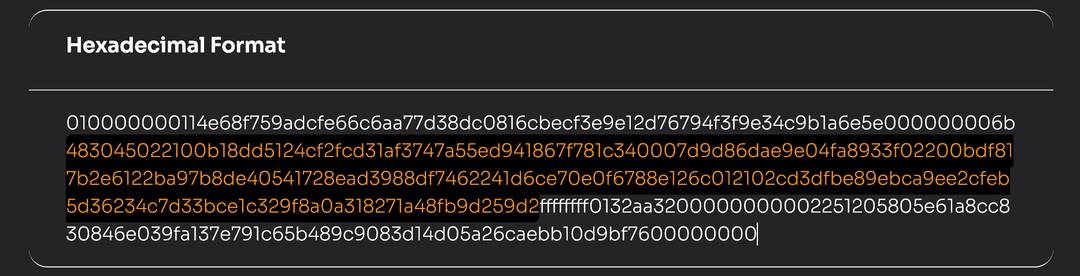
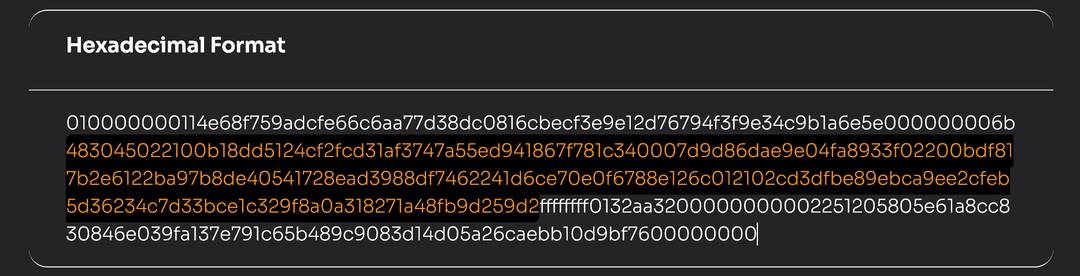
Let's break down the highlighted hex string from our transaction:
48 3045...126c01 21 02cd...259d2
Hex Value | Length | Description |
---|---|---|
48 | 1 byte | Signature length in hex (72 bytes in decimal) |
3045...126c01 | 71 bytes + 1 byte | DER-encoded signature (71 bytes) + SIGHASH_ALL byte (01) |
21 | 1 byte | Public key length in hex (33 bytes in decimal) |
02cd...259d2 | 33 bytes | Compressed public key (02 prefix indicates even y-coordinate) |
This ScriptSig follows the standard P2PKH (Pay to Public Key Hash) pattern, which we can identify by its two key components:
- A DER-encoded signature followed by SIGHASH byte (
3045...126c01
) - A compressed public key (
02cd...259d2
)
<signature> <pubkey>
The signature proves ownership of the private key, while the public key must hash to match the address in the previous output's ScriptPubKey.
1- Purpose and Structure
The ScriptSig serves as the "key" that unlocks the previous output's "lock" (ScriptPubKey). It typically contains:
- Digital signatures proving ownership
- Public keys or other data required by the previous output's script
For legacy transactions, the ScriptSig contains both the signature and any other data needed to satisfy the previous output's spending conditions. For segwit transactions, most of this data moves to the witness field, leaving the ScriptSig empty or minimal.
2- Implementation Example
Here's how you might parse a ScriptSig:
def parse_scriptsig(raw_tx: bytes, offset: int = 0) -> tuple[bytes, int]:
"""
Parse a ScriptSig from raw transaction bytes
Args:
raw_tx: Raw transaction bytes
offset: Starting position in bytes
Returns:
(scriptsig, new_offset)
"""
# Read the script size (varint)
script_size, offset = read_varint(raw_tx, offset)
# Read the actual script bytes
script = raw_tx[offset:offset + script_size]
return script, offset + script_size
def decode_der_signature(sig_bytes: bytes) -> tuple[int, int]:
"""
Decode a DER-encoded ECDSA signature into r and s values
""" # Skip sequence byte
pos = 1 # Skip length byte
pos += 1 # Skip marker for r value
pos += 1 # Get r length
r_len = sig_bytes[pos]
pos += 1 # Get r value
r = int.from_bytes(sig_bytes[pos:pos + r_len], 'big')
pos += r_len # Skip marker for s value
pos += 1 # Get s length
s_len = sig_bytes[pos]
pos += 1 # Get s value
s = int.from_bytes(sig_bytes[pos:pos + s_len], 'big')
return r, s
Note
The ScriptSig format varies depending on the type of transaction. This example shows a P2PKH (Pay to Public Key Hash) input, which is the most common type.
3- Common Script Types
P2PKH (Pay to Public Key Hash)
The most common legacy transaction type has this ScriptSig pattern:
<signature> <pubkey>
P2SH (Pay to Script Hash)
For P2SH inputs, the ScriptSig contains:
<signatures...> <redeem script>
Segwit Transactions
For native segwit transactions (P2WPKH/P2WSH), the ScriptSig is empty as the unlocking data moves to the witness field.
Note
For P2SH-wrapped segwit transactions, the ScriptSig contains only the witness program, while the actual unlocking data is in the witness field.
4- Size Considerations
The ScriptSig size is encoded as a varint before the actual script data. Common sizes are:
- P2PKH: ~107 bytes
- P2SH: Variable (depends on redeem script complexity)
- Native Segwit: 0 bytes
- P2SH-wrapped Segwit: ~23 bytes
Warning
Large ScriptSigs increase transaction size and therefore fees. Segwit moves this data to the witness, which receives a fee discount.
5- Validation Rules
When validating a ScriptSig:
- The script must parse successfully
- The combined script (ScriptSig + ScriptPubKey) must execute without errors
- The final stack must contain a true value
- The script size must not exceed the maximum allowed size
Historical Note
The original Bitcoin design allowed more complex operations in ScriptSig, but many were disabled to prevent transaction malleability. This led to the development of segregated witness (segwit).