Outputs: Output count
Size
variable
Format
Compact Size
Description
Number of outputs in this transaction.
Example
02
Byte Visualization
The number of outputs in a transaction is stored as a variable-length integer (varint). Let's examine a real transaction:
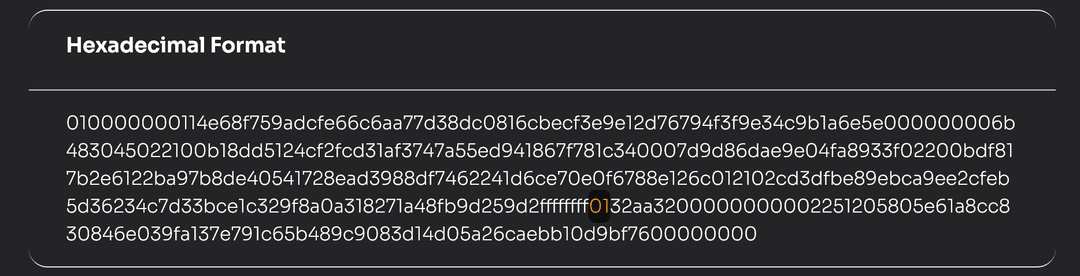
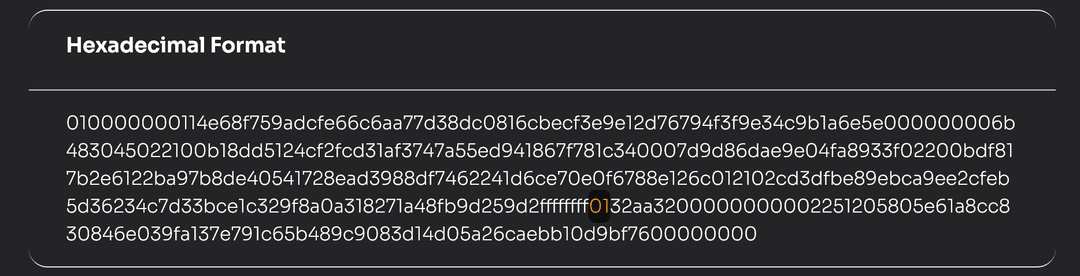
The output count tells us how many outputs to expect in this transaction. Like the input count, it uses varint encoding:
Decimal Range | Hex Range | Bytes used | Format |
---|---|---|---|
0 to 252 |
| 1 | uint8_t |
253 to 2¹⁶-1 |
| 3 |
|
2¹⁶ to 2³²-1 |
| 5 |
|
2³² to 2⁶⁴-1 |
| 9 |
|
Common Output Patterns
Most Bitcoin transactions have either one or two outputs:
- Single Output: Typically seen in coinbase transactions (mining rewards) or when moving all funds to a new address
- Two Outputs: The most common pattern, where one output is the payment and the other is change returned to the sender
- Multiple Outputs: Used for batching multiple payments into a single transaction, which is more efficient
Implementation Example
Here's how you might parse a transaction's output count:
def parse_output_count(raw_tx: bytes, offset: int = 0) -> tuple[int, int]:
"""
Parse output count from raw transaction bytes
Args:
raw_tx: Raw transaction bytes
offset: Starting position in bytes
Returns:
(count, new_offset)
"""
# Read the varint
count = 0
first_byte = raw_tx[offset]
if first_byte < 0xfd:
count = first_byte
offset += 1
elif first_byte == 0xfd:
count = int.from_bytes(raw_tx[offset+1:offset+3], 'little')
offset += 3
elif first_byte == 0xfe:
count = int.from_bytes(raw_tx[offset+1:offset+5], 'little')
offset += 5
else: # 0xff
count = int.from_bytes(raw_tx[offset+1:offset+9], 'little')
offset += 9
return count, offset
Note
Every valid Bitcoin transaction must have at least one output. A transaction with zero outputs would be invalid and rejected by the network.