Locktime
Size
4 bytes
Format
Little-Endian
Description
The block number or timestamp at which this transaction is unlocked.
Example
00000000
Byte Visualization
The locktime field is a 4-byte value that specifies the earliest time a transaction can be added to the blockchain.
Let's examine our transaction to understand this better:
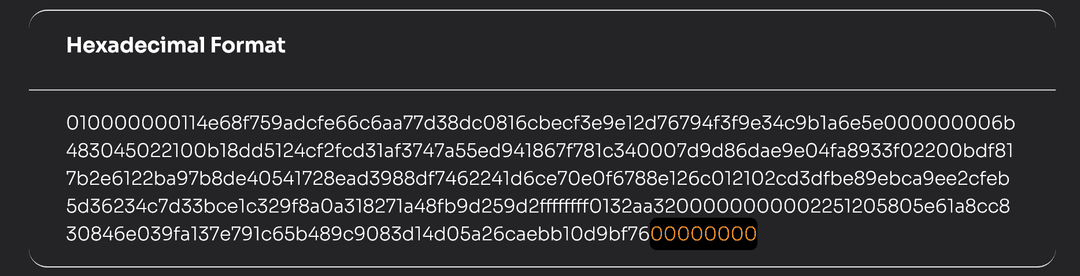
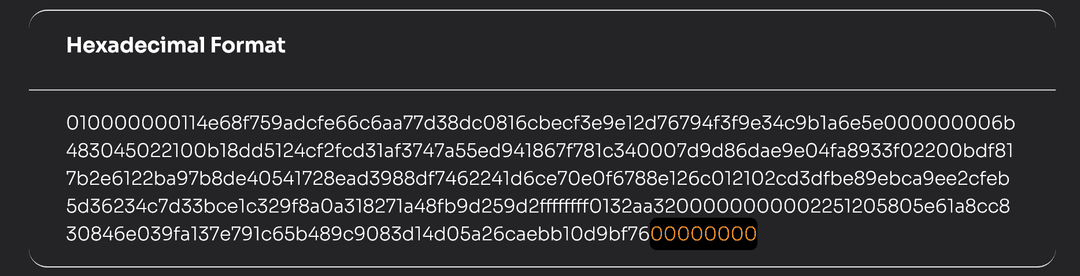
In our example transaction, we can see the locktime value is 00000000
. This means:
- The transaction is valid immediately (can be included in the next block)
- No timelock restrictions are applied
- This is the most common locktime value used in Bitcoin transactions
Locktime Values
The interpretation of the locktime value depends on its range:
Locktime Value | Interpretation |
---|---|
0 | Transaction is valid immediately (most common) |
< 500.000.000 | Interpreted as a block height |
≥ 500.000.000 | Interpreted as a Unix timestamp |
For example:
- Locktime of 650000 means the transaction is invalid until block 650000
- Locktime of 1640995200 means the transaction is invalid until January 1, 2022 (Unix timestamp)
Note
Locktime is only enforced if at least one input has a sequence number below 0xffffffff. If all inputs have sequence 0xffffffff, the locktime is ignored.
Implementation Example
Here's how you might parse a transaction's locktime:
def parse_locktime(raw_tx: bytes, offset: int = 0) -> tuple[int, int]:
"""
Parse locktime from raw transaction bytes
Args:
raw_tx: Raw transaction bytes
offset: Starting position in bytes
Returns:
(locktime, new_offset)
"""
# Read 4 bytes for locktime
locktime_bytes = raw_tx[offset:offset + 4]
# Convert to integer (little-endian)
locktime = int.from_bytes(locktime_bytes, 'little')
return locktime, offset + 4
Use Cases
1. Time-Delayed Transactions
Locktime can be used to create transactions that only become valid in the future:
def create_future_tx(block_height: int) -> bytes:
"""Create a transaction valid only after specific block height"""
tx = Transaction()
# ... add inputs and outputs ...
# Set locktime to future block
tx.locktime = block_height
# Must set sequence < 0xffffffff for locktime to be enforced
for tx_in in tx.inputs:
tx_in.sequence = 0xfffffffe
return tx.serialize()
2. Escrow Transactions
Locktime can be used in escrow services where funds should only be released after a certain time:
def create_escrow_tx(release_time: int) -> bytes:
"""Create a transaction that releases funds at specific time"""
tx = Transaction()
# ... add inputs and outputs ...
# Set locktime to future timestamp
tx.locktime = release_time
# Enable locktime enforcement
for tx_in in tx.inputs:
tx_in.sequence = 0xfffffffe
return tx.serialize()
Historical Note
Locktime was originally intended to be used with sequence numbers for
"high-frequency trades" - a way to update transactions before they were mined.
Validation Rules
When validating a transaction with locktime:
- If all input sequences are 0xffffffff, locktime is ignored
- Otherwise:
- If locktime < 500000000: Compare against current block height
- If locktime ≥ 500000000: Compare against median time past (MTP)
- Transaction is invalid if current time/height is less than locktime
Warning
Using locktime for time-sensitive applications requires careful consideration of block time variance and potential network reorganizations.